Introduction:
Welcome to the world of C programming! Whether you’re a complete novice or looking to enhance your coding skills, this guide will walk you through the fundamentals and beyond. C is a powerful and versatile programming language widely used for system-level programming, embedded systems, and application development. Let’s dive in!
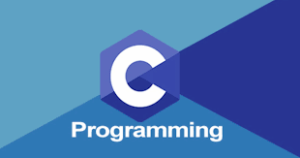
Chapter 1: Understanding the Basics of C Programming
In this chapter, we’ll cover the fundamental concepts that form the backbone of C programming.
- What is C?
- C is a general-purpose, procedural programming language developed by Dennis Ritchie at Bell Labs in the early 1970s. It is known for its efficiency, flexibility, and close-to-the-hardware capabilities.
- Setting Up Your C Environment
- Before writing C code, you need a development environment. Learn to set up a C compiler like GCC and a text editor or an integrated development environment (IDE) such as Visual Studio Code.
- Your First C Program
- Dive right in by creating a simple “Hello, World!” program. Understand the basic structure of a C program, including headers, main functions, and statements.
Chapter 2: Variables, Data Types, and Operators
- Variables in C
- Explore the concept of variables and containers that store data values. Learn about naming conventions, data types, and declarations.
- Data Types in C
- Understand different data types such as int, float, char, and more. Know the significance of choosing the right data type for efficient memory usage.
- Operators in C
- Delve into arithmetic, relational, logical, and assignment operators. Comprehend their use in various operations and expressions.
Chapter 3: Control Flow and Decision Making
- Conditional Statements
- Explore if, else if, and else statements for making decisions in your program based on conditions.
- Switch Statements
- Learn about switch statements as an alternative to multiple if-else conditions, improving code readability.
- Loops in C
- Understand for, while, and do-while loops for repetitive execution of code. Grasp the concept of loop control statements.
Chapter 4: Functions in C
- Introduction to Functions
- Learn about functions, a way to break down your code into modular and reusable components.
- Function Prototypes and Definitions
- Understand how to declare function prototypes and define functions. Explore the concept of function parameters and return values.
- Recursion
- Delve into recursive functions, where a function calls itself. Understand their application and potential pitfalls.
Chapter 5: Arrays and Strings
- Arrays in C
- Explore one-dimensional and multi-dimensional arrays. Understand array indexing, initialization, and manipulation.
- Strings in C
- Learn about the C string library, handling strings as character arrays, and common string manipulation functions.
Chapter 6: Pointers and Memory Management
- Understanding Pointers
- Grasp the concept of pointers, variables that store memory addresses. Explore pointer arithmetic and dynamic memory allocation.
- Memory Management
- Learn about malloc(), free(), calloc(), and realloc() functions for dynamic memory management.
Chapter 7: File Handling in C
File Input and Output
- Understand file handling in C. Learn how to open, read, write, and close files.
Here’s a simple C program that prints “Hello, World!” to the console, which is often the first program written when learning a new programming language:
Program that Prints:
Here’s a simple C program that prints “Hello, World!” to the console, which is often the first program written when learning a new programming language:
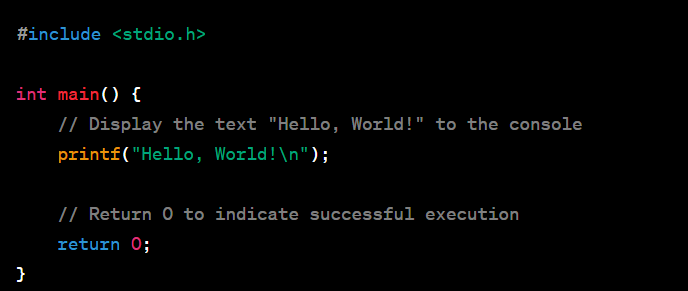
This program includes the standard input/output library (#include <stdio.h>
) for basic input and output functions. The main
function is the entry point of the program, and within it, the printf
function is used to print the text “Hello, World!” to the console. The \n
at the end represents a newline character, which moves the cursor to the next line.
C language Code:
1. Input and Output:
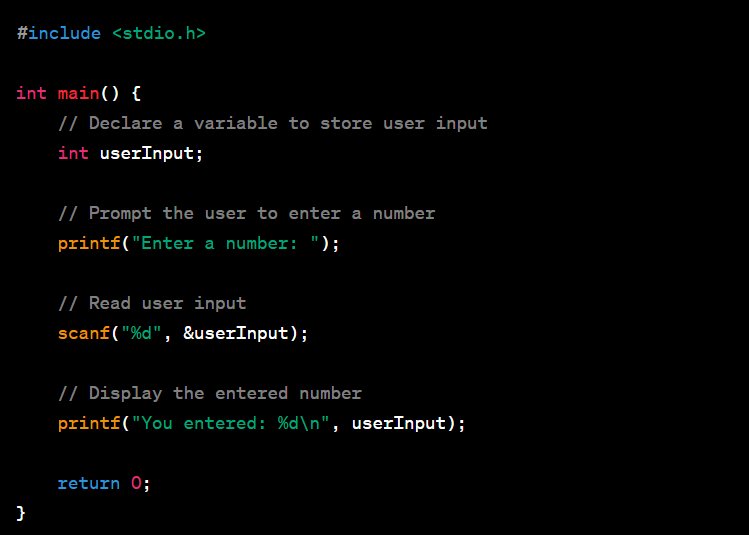
This program prompts the user to enter a number, reads the input, and then prints the entered number.
2. Conditional Statements:
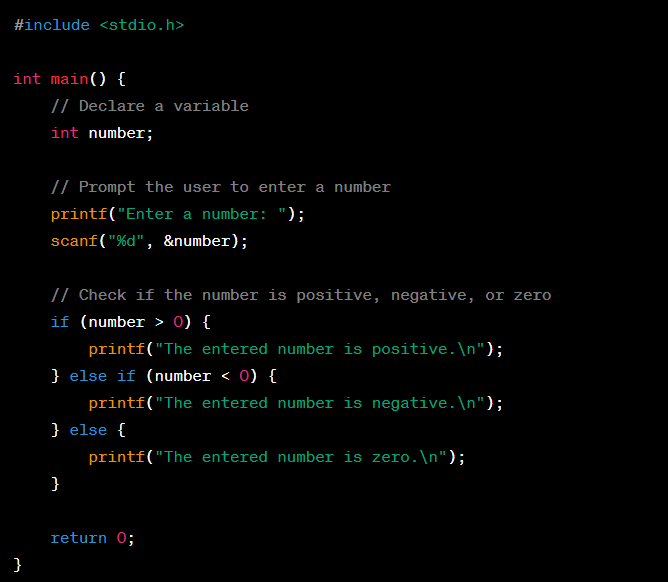
This program uses conditional statements (if
, else if
, else
) to check if the entered number is positive, negative, or zero.
3. Loops:
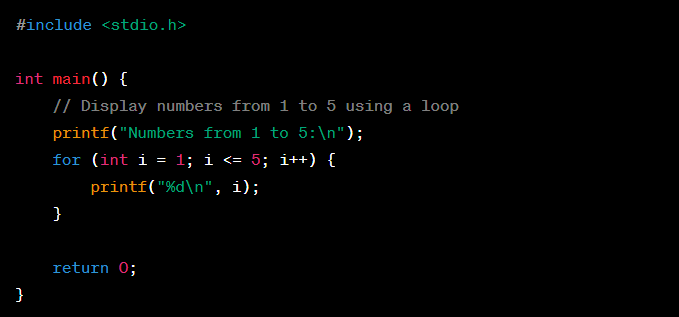
This program uses a for
loop to display numbers from 1 to 5.
4. Functions:
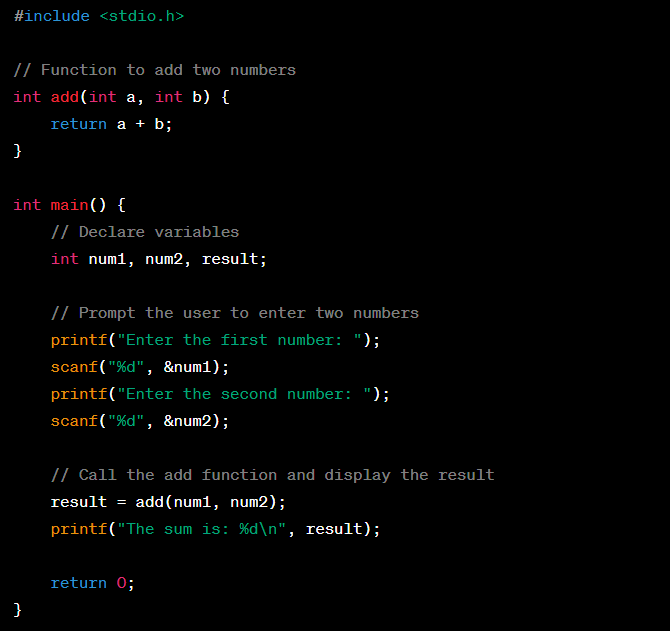
This program defines a simple function add
that takes two parameters and returns their sum. The main
function prompts the user to enter two numbers, calls the add
function, and prints the result.
Conclusion:
Congratulations on completing this comprehensive guide to C programming! You now have a solid foundation to explore advanced topics, such as data structures, and algorithms, and even dive into system-level programming. Continuously practice, build projects, and explore the vast world of C to become a proficient programmer. Happy coding!